How To Set The Rotation Of An Object In Unity
Matt Bird writes all sorts of nonsense, but he enjoys writing tutorials for Unity.
In that location are many reasons yous might rotate an object in Unity. Maybe a door needs to swing on a pivot indicate, or an enemy needs to spin in a particular fashion as they fly through the air, or, virtually likely, a character may need to turn when moved. Rotation is useful for 2D and 3D games alike, and having at least a grounding in how to practice it is wise.
There are, in general, 3 different ways to rotate an object in Unity.
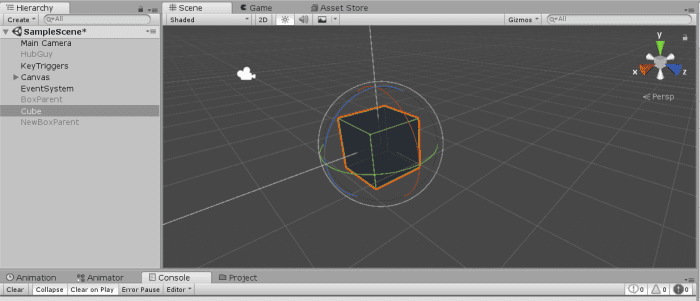
Screenshots taken by myself. Unity owned and developed by Unity Technologies.
Method ane: Rotate in Scene View
Have a expect at the screenshot above. Here we have an object on a 3D plane in the Scene View window. Normally when you lot select an object, it will have guidance arrows that let you to move it around, only right at present, it has iii rings around information technology. If you click on the Rotate Tool in the top-left corner of the screen - it's a pair of arrows formed into a circumvolve - the rings volition appear, allowing yous to rotate the object freely. Gear up information technology nevertheless you like.
The red ring volition change the object'south x-axis orientation; the greenish band volition modify the object's y-axis orientation, and the blue band will change the object's z-centrality orientation.
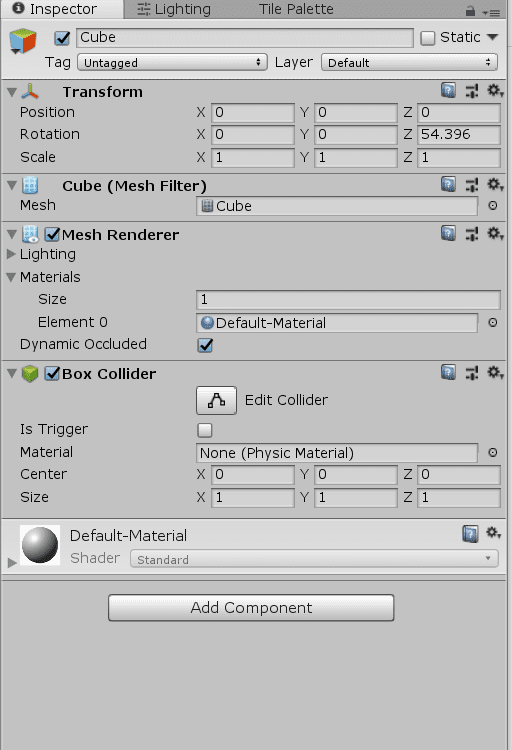
Method 2: Rotate via the Inspector
The second method is to change the GameObject'due south Rotation values. Click on the object and look at the Inspector on the right. The acme component, Transform, has three Rotation values for x, y, and z. Inbound numbers into these fields will alter the rotation of the GameObject in the Scene view.
(Messing with the rotation of an object tin get messy at times, so if you ever desire to showtime from square i, simply change all iii values to zero.)
While we are in the Inspector, information technology is worth noting that these values only capture the rotation of the GameObject on a global perspective. If the GameObject is childed to another GameObject its Rotation values reverberate its rotation relative to its parent. So if the parent is rotated and the kid object is rotated with information technology, the child'due south Rotation values will initially announced every bit 0, 0, and 0.
The following 2 methods are only useful for rotating GameObjects outside of runtime. If yous want to rotate objects during runtime, yous'll need to exercise some coding.
Method 3: Rotate via Code
A simple rotation effect is pretty easy to reach while programming and requires but a unmarried line of lawmaking to put into event. Information technology goes like this:
Coil to Go along
if(Input.GetKeyDown(KeyCode.B)) { itemToRotate.transform.Rotate(45f, 45f, 0f, Space.Self); }
In this instance, we take a GameObject, itemToRotate, and we want to gear up it at 45-degree angles. We access the GameObject's Transform and use the Rotate role to set its 3 euler values. At that place is also a setting for the GameObject's relativity, which brings us back to local and world space. If y'all want the object to rotate relative to the world, use Space.Earth; if yous desire the object to rotate relative to itself, employ Space.Self.
This code volition quickly and painlessly rotate the GameObject. In most cases, nonetheless, this is not the lawmaking you want to apply, because it is instantaneous. In club to see the GameObject actively rotate, you'll desire to try something like this:
private void Update() { if (Input.GetKeyDown(KeyCode.B)) { rotateObjectBool = truthful; } if(rotateObjectBool) { rotationTime = 180f; itemToRotate.transform.Rotate(Vector3.down * (rotationTime * Time.deltaTime)); } }
Here nosotros apply a simple bool trigger, rotateObjectBool, to set the rotation in motion. When the thespian hits the B central itemToRotate begins to rotate along a Vector3 over time. Time.deltatime measures the amount of time since the final frame, and then multiplying a value by Time.deltatime will requite you a prissy, existent-time rotation. Changing the rotationTime variable will speed up (higher value) or slow downward (lower value) the rotation speed.
Vector3.down will decrease the object'southward y value over time. if you use Vector3.upward instead it will increase the object'due south y value and spin it in the opposite direction. Vector3.right and Vector3.left have a similar event on the object's x value. If you desire you can have lines that bear upon both the x and y values simultaneously, making for a slap-up spinning event.
These Vector3 variables are all well and good when yous desire an object to spin freely forever. Update will proceed them going until the game stops, or, in this case, until the bool is changed to faux.
But what if you want a GameObject to rotate to a specific betoken and and then terminate? There are many ways to practise this, but for this case, we will use a Coroutine. Coroutines are functions that carry out code over time and tin be stopped at a designated signal. Nosotros'll apply a Coroutine to create the rotation outcome without Update:
private void Update() { if (Input.GetKeyDown(KeyCode.B)) { StartCoroutine(RotateObject()); } } IEnumerator RotateObject() { bladder moveSpeed = 50f; Quaternion endingAngle = Quaternion.Euler(new Vector3(45, 45, 0)); while (Vector3.Distance(itemToRotate.transform.rotation.eulerAngles, endingAngle.eulerAngles) > 0.01f) { itemToRotate.transform.rotation = Quaternion.RotateTowards(itemToRotate.transform.rotation, endingAngle, moveSpeed * Time.deltaTime); yield return null; } itemToRotate.transform.rotation = endingAngle; }
We outset information technology off by hitting the B key. This triggers the StartCoroutine code, with the RotateObject() Coroutine equally its target. Coroutines always require 'StartCoroutine' earlier they will trigger, so don't forget this vital piece of code.
One time the IEnumerator is triggered, information technology sets two local variables into play. moveSpeed dictates the speed of rotation, while the Quaternion endingAngle determines the desired angles you want the GameObject to accomplish. (In near cases, you will set the values of endingAngle exterior the Coroutine, but this example is getting complicated enough every bit it is, so we'll go along it simple.)
Adjacent up is the while() statement. while() acts as a sort of local Update(), executing code independent within information technology continuously until the conditions for stopping have been met. In this case while() volition execute until itemToRotate's Rotation values are 0.01f unlike than those of endingAngle, using Distance to continuously calculate the divergence between these values. While executing itemToRotate'due south Rotation values will utilize Quaternion.RotateTowards, which gradually adjusts the x and y values until they are almost - only not quite exactly - what you desire.
(yield return null; dictates how long while volition await before it executes the rotation change again. Leaving it at 'zip' volition give you a smoothen, continuous plough.)
RotateTowards will never reach the exact values you desire, and then once the while() argument gets close enough it will break off. We and so set itemToRotate's Rotation values to those of endingAngle, resulting in nice, clean values rather than a bunch of decimals. The change in values is and then pocket-sized that there is no perceptible movement on screen. At that place! Object rotated.
Again, there are many ways to achieve this same effect, and results will vary depending on the path you accept. As a small-scale substitution, you lot tin try 'Quaternion.Lerp' or 'Quaternion.Slerp' instead of RotateTowards, resulting in more than gradual rotations that tedious down the closer they get to their endpoints.
This content is authentic and true to the best of the author'due south knowledge and is not meant to substitute for formal and individualized advice from a qualified professional.
Source: https://turbofuture.com/computers/How-to-Rotate-Objects-in-Unity
0 Response to "How To Set The Rotation Of An Object In Unity"
Post a Comment